Introduction to Authentication and Authorization
In the realm of web applications, ensuring security is paramount. Two fundamental concepts that play a pivotal role in this domain are authentication and authorization. These terms might sound similar, but they serve distinct purposes in safeguarding sensitive data and controlling access to resources.
Understanding the Importance of Authentication and Authorization
Authentication is the process of verifying the identity of a user or entity, ensuring that they are who they claim to be. It's like presenting your ID card to gain access to a restricted area. Without proper authentication mechanisms, anyone could potentially impersonate another user, leading to unauthorized access and security breaches.
On the other hand, authorization is the process of determining what actions a user is allowed to perform within a system or application. Once a user's identity is confirmed through authentication, authorization kicks in to define the level of access they have based on their role, permissions, or attributes. This ensures that users only have access to the resources and functionalities that are relevant to their roles or privileges.
In the context of web development, understanding and implementing robust authentication and authorization mechanisms are essential for safeguarding sensitive user data, protecting against unauthorized access, and maintaining the integrity of the application.
Brief Overview of the MERN Stack
The MERN stack is a popular and powerful technology stack used for building full-stack web applications. It comprises four key technologies:
1. MongoDB: A NoSQL database that stores data in flexible, JSON-like documents.
2. Express.js: a minimalist and flexible Node.js web application framework that provides a robust set of features for building web and mobile applications.
3. React.js: A JavaScript library for building user interfaces, particularly single page applications (SPAs), developed by Facebook.
4. Node.js: A server-side JavaScript runtime environment that executes JavaScript code outside a web browser, allowing developers to build scalable network applications.
Together, these technologies form a cohesive and efficient ecosystem for developing modern web applications. By leveraging the MERN stack, developers can build feature-rich, responsive, and scalable web applications with ease.
Read More: https://www.kumarijob.com/blog/career-tips/mern-stack-training
Key Concepts of Authentication
Authentication serves as the first line of defense in securing web applications by verifying the identity of users or entities attempting to access the system. Here's an exploration of its key concepts:
Explaining Authentication and its Role in Securing Web Applications:
Authentication is the process of validating the identity of users or entities trying to access a system. It ensures that only authorized individuals can interact with the application, protecting sensitive data and resources from unauthorized access. By requiring users to authenticate themselves through various methods, such as passwords, biometrics, or cryptographic keys, web applications establish trust and accountability, mitigating the risk of malicious activities like unauthorized data access or identity theft.
Different authentication methods include:
There are several authentication methods available, each with its own set of advantages and limitations:
Username/Password: This traditional method requires users to enter a unique username and password combination to gain access. Pros include familiarity and ease of implementation. However, passwords can be vulnerable to brute force attacks or phishing attempts.
Biometric Authentication: Utilizing physical characteristics such as fingerprints, facial recognition, or iris scans for authentication. Biometric authentication offers strong security and user convenience but may require specialized hardware and could raise privacy concerns.
Two-Factor Authentication (2FA): Combining two different authentication factors, such as a password and a one-time code sent via SMS or generated by an authenticator app. 2FA enhances security by adding an extra layer of protection against unauthorized access, but it may introduce complexity for users.
OAuth and OpenID Connect: Protocols for delegating authentication to third-party identity providers (e.g., Google, Facebook). OAuth enables secure access to user data without exposing passwords, while OpenID Connect adds identity verification capabilities. These methods streamline the authentication process but require integration with external services and careful handling of access tokens.
Understanding Authorization
Authorization complements authentication by determining the level of access users have to resources within a system. Here's an exploration of its key concepts:
Defining Authorization and its Significance in Access Control:
Authorization is the process of granting or denying access to specific resources or functionalities based on a user's identity, role, or other attributes. It ensures that users can only perform actions that are permitted within the system, enforcing security policies and protecting sensitive data from unauthorized manipulation or disclosure. By defining granular access controls, web applications can maintain data integrity, confidentiality, and compliance with regulatory requirements.
There are two primary approaches to implementing authorization:
Role-based Access Control (RBAC): Assigning permissions to users based on predefined roles or groups. Users are granted access rights associated with their roles, simplifying access management, and ensuring consistency across the system. However, RBAC may lack flexibility in handling complex access scenarios or individualized permissions.
Attribute-based Access Control (ABAC): Dynamically evaluating access decisions based on a set of attributes associated with users, resources, and environmental conditions. ABAC offers fine-grained control over access policies, allowing for more nuanced authorization decisions tailored to specific contexts or conditions. However, implementing ABAC may require additional complexity in policy management and enforcement.
Understanding the nuances of authentication and authorization is crucial for building secure and robust web applications that protect user data and uphold privacy standards.
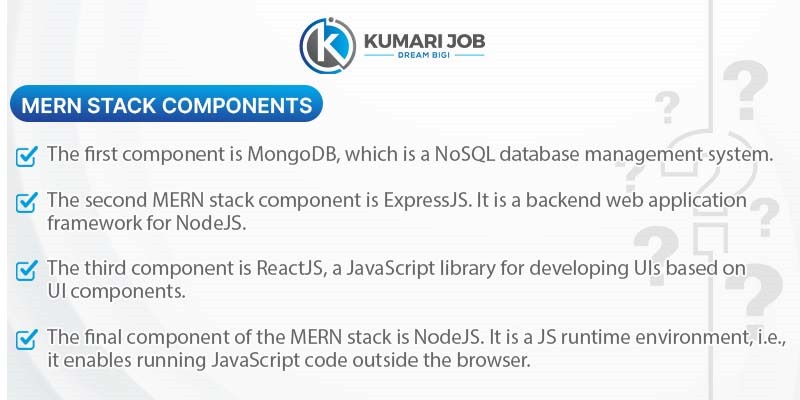
Setting Up the MERN Stack Environment
Setting up the MERN (MongoDB, Express.js, React.js, and Node.js) stack environment is the foundational step for building web applications. Here's how to get started:
Installing and Configuring MongoDB: Download and install MongoDB from the official website or package manager. Configure MongoDB by setting up a data directory and defining the necessary permissions. Start the MongoDB service to begin using the database.
Setting Up Express.js Server:Install Node.js, create a project directory, initialize it with npm init, install Express.js, set up a server, import Express, create an instance, and define routes and middleware.
Creating a React.js Frontend: Install the createreact app globally, create a new React.js project, navigate to the project directory, start the development server, and customize your application by modifying components, styles, and dependencies.
Integrating Node.js for Backend Functionality: Node.js comes with npm for managing backend code, creating APIs, and installing additional packages. It supports Express.js routes, MongoDB connection, and middleware for error handling and logging.
Setting up the MERN stack environment lays the groundwork for building feature-rich web applications with robust backend functionality and a dynamic frontend interface. As you progress, you can further customize and extend your application to meet specific requirements and deliver an exceptional user experience.
User Authentication with Passport.js
Passport.js is a popular authentication middleware for Node.js, widely used in the MERN stack ecosystem. Here's how to utilize Passport.js for user authentication:
Introduction to Passport.js for Authentication: Passport.js is a Node.js authentication library that supports various strategies like local username/password, OAuth, and OpenID, offering a streamlined, modular approach for easy integration with existing applications.
Implementing a Local Strategy for Username/Password Authentication: Install Passport.js and its dependencies using npm. Configure it in your Express.js application, initialize session management, define a local authentication strategy, implement user authentication logic, and serialize and deserialize user objects to maintain session state and facilitate user authentication across requests.
Integrating OAuth for Social Media Login:To use OAuth, install the passport OAuth and passport Google OAuth packages using npm. Register your application with an OAuth provider like Google or Facebook to obtain client credentials. Configure Passport.js to use the OAuth authentication strategy, implement route handlers, retrieve user profile information, and manage user sessions and authentication tokens to maintain user authentication state across requests.
User authentication with Passport.js allows you to implement secure authentication mechanisms in your MERN stack application, whether using traditional username/password credentials or integrating social media login functionality via OAuth. By leveraging Passport.js's flexibility and extensibility, you can provide users with a seamless and secure authentication experience while ensuring the integrity and confidentiality of their credentials.
JWT Authentication
JWT (JSON Web Tokens) authentication is a popular approach for securing web applications in the MERN stack. Here's how to implement JWT authentication:
Introduction to JSON Web Tokens (JWT): JSON Web Tokens (JWTs) are URL-safe tokens used for authentication and authorization, consisting of a header, payload, and signature, encoded as Base64 strings separated by periods.
Generating and Verifying JWT Tokens: To generate JWT tokens, use a secret key known only to your application and use Node.js libraries like `JSON web tokens`. Authenticate a user, generate a token with user information, sign it with the secret key, and verify its signature using the same key to ensure it's not expired or tampered with.
Implementing JWT Authentication in MERN Stack: Install the `JSON web token` library in your Node.js backend, implement authentication middleware for JWT token verification, generate a token with user information, store it securely, include it in HTTP requests, verify it on the server side, implement token expiration and refresh mechanisms, and handle token renewal or reauthentication.
JWT authentication provides a stateless and scalable approach to user authentication in MERN stack applications. By leveraging JWT tokens, you can implement secure authentication mechanisms while simplifying session management and improving performance.
User Registration and Login
User registration and login functionalities are fundamental aspects of any web application. Here's how to design and implement them in a MERN stack application:
Designing and Implementing User Registration Forms: Create a user registration form with user information, implement client-side validation, use React.js for a user-friendly interface, implement backend API endpoints for registration, and securely store user passwords using libraries.
Handling User Login and Authentication Requests: This document outlines the process of creating a login form with fields for username, email, and password, implementing client-side validation, sending a login request to the backend server, validating user credentials on the server side, and generating a JWT token with user information. It also discusses serverside validation using libraries like Express Validator in Express.js, generating a JWT token with a secret key, signing it to prevent tampering, sending it back to the client, and securely storing the token for future authenticated requests.
User registration and login functionalities are critical for enabling users to access protected resources and personalize their experience within the application. By following best practices for user authentication and session management, you can ensure a secure and seamless user authentication process in your MERN stack application.
Introduction to Two-Factor Authentication (2FA):
Two-factor authentication (2FA) is a security mechanism that requires users to provide two distinct authentication factors to verify their identity, with the first being a known password and the second being a possessed item like a mobile device or security token.
Integrating 2FA for Enhanced Security: Choose the right 2FA method for your application, such as time-based one-time passwords (TOTP), SMS-based OTPs, or hardware tokens. Implement serverside logic to enable 2FA during user registration and account setup, securely store 2FA-related information in a database, and provide clear instructions on how to use 2FA.
Using authenticator apps or SMS for 2FA: Authenticator apps, like Google Authenticator or Authy, allow users to generate and link a QR code with their account for TOTP-based authentication. SMS-based authentication involves collecting and verifying users' mobile numbers, sending one-time codes, and prompting them to enter the received OTP.
Implementing two-factor authentication provides an additional layer of security to your MERN stack application, enhancing protection against unauthorized access and safeguarding sensitive user data. By integrating 2FA methods such as authenticator apps or SMS-based authentication, you can strengthen the security posture of your application while maintaining usability and the user experience.
Compliance and Regulations
Compliance with data privacy regulations is crucial for ensuring the protection of user data and maintaining trust in your MERN stack application. Here's how to navigate key regulations and implement features for compliance:
Understanding GDPR, CCPA, and Other Data Privacy Regulations:
The General Data Protection Regulation (GDPR) and the California Consumer Privacy Act (CCPA) are EU-enforced data privacy laws, focusing on transparency, data minimization, purpose limitation, and accountability. Other data privacy laws include the PIPEDA in Canada and the PDPA in Singapore, each with unique requirements and provisions.
Implementing Features for Compliance with Data Protection Laws:
The text emphasizes the importance of data minimization, user rights, data security, and privacy policies in a user-friendly application. It emphasizes the need for data minimization, user rights, robust security measures, and clear, understandable privacy policies to protect sensitive information and ensure user accessibility. It also highlights the need for robust security measures.
Ensuring User Consent and Data Transparency:
Consent management involves obtaining explicit user consent before data collection, processing, or sharing, and providing granular consent options. Transparency and accountability involve being transparent about data practices, maintaining records, and regularly reviewing and updating data protection policies.
Compliance with data privacy regulations is an ongoing effort that requires diligence, proactive measures, and a commitment to protecting user privacy and rights. By understanding relevant regulations, implementing appropriate features and controls, and fostering transparency and accountability, you can build trust with users and demonstrate your commitment to data protection in your MERN stack application.
Troubleshooting Common Issues
Troubleshooting is an integral part of maintaining and optimizing a MERN stack application. Here's how to address common issues related to authentication and authorization:
Debugging authentication and authorization failures:
The text emphasizes the importance of reviewing server logs, error messages, and authentication middleware to identify root causes of authentication or authorization failures, verifying user credentials validation, using logging and debugging tools, and implementing error handling mechanisms.
Handling common issues like token expiration, CORS errors, etc.:
Token expiration policies and handling of CORS errors are crucial for JWT users. Server settings should allow requests from specified origins, and CORS headers should be correctly set. Addressing network connectivity, server downtime, database outages, monitoring performance, and staying informed about security vulnerabilities are also essential.
Seeking community support and resources for problem-solving:
Join online communities, and Q&A platforms, consult documentation, and attend webinars, workshops, and conferences for MERN stack development. Consider hiring experienced developers or consultants for guidance and troubleshooting complex issues.
By proactively addressing common issues and leveraging community support and resources, you can effectively troubleshoot and resolve challenges encountered during the development and operation of your MERN stack application.
Read More: https://www.kumarijob.com/blog/career-tips/mern-stack
Frequently Asked Questions
Kumari Job, Broadway Infosys, Mindrisers, etc provides MERN Stack training in Nepal.
It depends on your basic skills. If you already know languages like HTML, CSS, and JavaScript, you can become a beginner MERN stack developer in about 8-10 weeks with regular hands-on practice.
Web development in 2024 has changed substantially, but the demand for MERN and MEAN stacks continues to be present. The immense popularity of these stacks in the scene of web development in 2024 is due to several factors, such as robustness, the evolving ecosystem of JavaScript, and the scalability factor.
MERN, known for its JavaScript-based full-stack development, provides a seamless and efficient environment for building modern web applications. On the other hand, PHP, a stalwart in web development for decades, offers server-side scripting capabilities with a wide range of frameworks and applications
Conclusion
Mastering authentication and authorization mechanisms is imperative for ensuring the security and integrity of web applications, particularly within the context of the MERN stack. Through this comprehensive guide, we've delved into the intricacies of these essential concepts, providing insights into their importance, implementation strategies, and best practices.
By understanding the fundamentals of authentication and authorization, as well as leveraging powerful tools and frameworks like Passport.js and JWT authentication, developers can fortify their MERN stack applications against potential threats and vulnerabilities. Additionally, compliance with data privacy regulations underscores the significance of safeguarding user data and fostering transparency in data practices. Troubleshooting common issues is a critical aspect of maintaining a robust MERN stack application. By adopting proactive troubleshooting strategies and tapping into community support and resources, developers can effectively address challenges and ensure the seamless operation of their applications.